파드 세트에 대한 논리적 추상화이다.
안정적인 단일 엔드포인트를 통해 노출한다.
Demo
sec04의 1번을 복사한다.
# 01-simple-deploy.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deploy
spec:
selector:
matchLabels:
app: my-app
replicas: 3
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: nginx
image: nginx
ports:
- containerPort: 80
이후 service 파일을 생성한다.
# 02-simple-service.yaml
apiVersion: v1
kind: Service
metadata:
name: nginx
spec:
selector:
app: my-app
ports:
- port: 80
targetPort: 80
kubectl apply -f .
적용 후 세부 사항을 살펴보자
kubectl describe svc nginx
#결과
Name: nginx
Namespace: default
Labels: <none>
Annotations: <none>
Selector: app=my-app
Type: ClusterIP
IP Family Policy: SingleStack
IP Families: IPv4
IP: 10.96.192.111
IPs: 10.96.192.111
Port: <unset> 80/TCP
TargetPort: 80/TCP
Endpoints: 10.244.1.9:80,10.244.2.10:80,10.244.1.10:80
Session Affinity: None
Internal Traffic Policy: Cluster
Events: <none>
올라가 있는 파드를 지우거나 늘리거나 변경되면 Endpoints가 즉시 업데이트 된다.
Load Balancing
#03-svc-load-balancing.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deploy
spec:
selector:
matchLabels:
app: my-app
replicas: 3
template:
metadata:
labels:
app: my-app
spec:
terminationGracePeriodSeconds: 1
containers:
- name: nginx
image: vinsdocker/nginx-gke
ports:
- containerPort: 80
---
apiVersion: v1
kind: Service
metadata:
name: my-app
spec:
selector:
app: my-app
ports:
- port: 80
targetPort: 80
---
apiVersion: v1
kind: Pod
metadata:
name: demo-pod
spec:
terminationGracePeriodSeconds: 1
containers:
- name: demo
image: vinsdocker/util
args:
- "sleep"
- "3600"
kubectl apply -f 03-svc-load-balancing.yaml.yaml
클러스터 내부에서 파드끼리 통신하기 위한 demo-app과 로드밸런싱을 위한 service, my-app을 만든다.
이후 pod 내부에 접속한다.
kubectl exec -it demo-pod -- bash
이 후 요청을 보내보면 매번 다른 pod 이름을 보여주는것을 확인할 수 있다.
# 반복
root@demo-pod:/# curl my-app
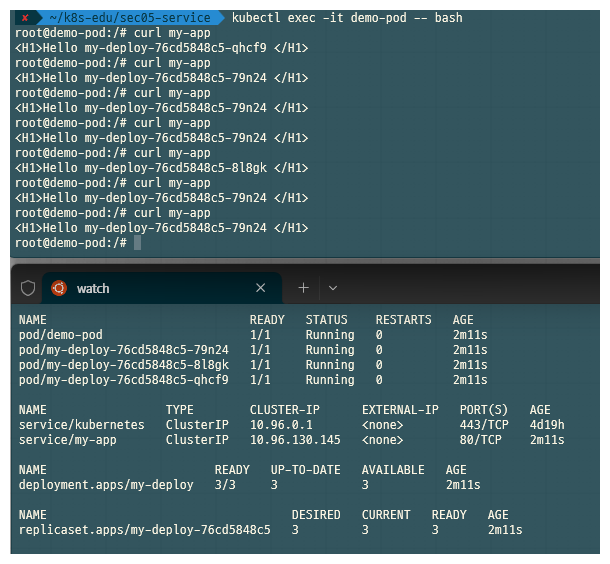
Service Types
타입 | 설명 |
ClusterIP | K8S 클러스터 내부에서 통신을 위한 타입이다. 클러스터 외부에서는 접근할 수 없다. 타입을 지정하지않으면 기본값이다. |
NodePort | 클러스터 외부에서 K8S 마스터/노드를 통해 특정 포트를 사용해서 접근할 수 있다. |
LoadBalancer | 외부에서 들어오는 트래픽을 수신하는데 사용된다. (AWS, GCP 같은 클라우드 업체에서 사용된다.) |
* 클러스터 외부의 노출은 ingress를 사용하며 이는 나중에 학습한다.
Kind cluster with extra port mapping
기존 클러스터를 삭제하고 추가 포트매핑을 한 클러스터를 생성해보자
kind delete cluster --name dev-cluster
아래처럼 파일을 생성하고 클러스터를 생성하자
kind: Cluster
apiVersion: kind.x-k8s.io/v1alpha4
name: dev-cluster
nodes:
- role: control-plane
extraPortMappings:
- containerPort: 30001
hostPort: 30001
protocol: TCP
- role: worker
- role: worker
kind create cluster --config [yaml 파일]
아래 명령어를 통해 port 매핑을 확인할 수 있다.
docker ps -a
# 결과
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS
NAMES
6e4af28b723c kindest/node:v1.31.2 "/usr/local/bin/entr…" 31 seconds ago Up 28 seconds 0.0.0.0:30001->30001/tcp, 127.0.0.1:45971->6443/tcp dev-cluster-control-plane
056d4e90bc99 kindest/node:v1.31.2 "/usr/local/bin/entr…" 31 seconds ago Up 28 seconds
dev-cluster-worker
76da068e1786 kindest/node:v1.31.2 "/usr/local/bin/entr…" 31 seconds ago Up 28 seconds
dev-cluster-worker2
Nodeport - Demo
# 05-nodeport-service.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deploy
spec:
selector:
matchLabels:
app: my-app
replicas: 3
template:
metadata:
labels:
app: my-app
spec:
terminationGracePeriodSeconds: 1
containers:
- name: nginx
image: vinsdocker/nginx-gke
ports:
- containerPort: 80
---
apiVersion: v1
kind: Service
metadata:
name: my-app
spec:
type: NodePort
selector:
app: my-app
ports:
- port: 80
targetPort: 80
nodePort: 30001
kubectl apply -f 05-nodeport-service.yaml
watch 명령어로 확인해보면 NodePort가 있는것을 확인할 수 있고
아래 페이지로 들어가보면 접속이 되는것을 확인할 수 있다.
http://localhost:30001/
Rolling Update With Service
# 06-rolling-update-demo.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: order-service-deployment
spec:
minReadySeconds: 5
strategy:
type: RollingUpdate
rollingUpdate:
maxSurge: 0
maxUnavailable: 1
selector:
matchLabels:
app: order-service
replicas: 3
template:
metadata:
labels:
app: order-service
spec:
containers:
- name: order-service-container
image: vinsdocker/k8s-app:v1
ports:
- name: "app-port"
containerPort: 80
---
apiVersion: v1
kind: Service
metadata:
name: my-app
spec:
selector:
app: order-service
ports:
- port: 80
targetPort: 80
---
apiVersion: v1
kind: Pod
metadata:
name: demo-pod
spec:
terminationGracePeriodSeconds: 1
containers:
- name: demo
image: vinsdocker/util
args:
- "sleep"
- "3600"
kubectl apply -f 06-rolling-update-demo.yaml
위 파일을 생성하고 접속한다.
kubectl exec -it demo-pod -- bash
이후 아래 명령어를 쳐보면 V1이 나오는것을 확인할 수 있다.
for i in {1..1000}; do curl -s http://my-app | grep -o "<title>[^<]*" | tail -c+8; done
이때 빠르게 v2로 변경하고 적용하면 V1과 V2가 섞여서 나오는것을 확인할 수 있다.
그러다가 모두 V2로 변경되는것을 확인할 수 있다.
'Kubernetes' 카테고리의 다른 글
[Kubernetes] Probes (0) | 2024.12.19 |
---|---|
[Kubernetes] Namespace (0) | 2024.12.19 |
[Kubernetes] Deployment (0) | 2024.12.16 |
[Kubernetes] ReplicaSet (0) | 2024.12.12 |
[Kubernetes] Pod 기초 - 2 (1) | 2024.12.06 |
파드 세트에 대한 논리적 추상화이다.
안정적인 단일 엔드포인트를 통해 노출한다.
Demo
sec04의 1번을 복사한다.
# 01-simple-deploy.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deploy
spec:
selector:
matchLabels:
app: my-app
replicas: 3
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: nginx
image: nginx
ports:
- containerPort: 80
이후 service 파일을 생성한다.
# 02-simple-service.yaml
apiVersion: v1
kind: Service
metadata:
name: nginx
spec:
selector:
app: my-app
ports:
- port: 80
targetPort: 80
kubectl apply -f .
적용 후 세부 사항을 살펴보자
kubectl describe svc nginx
#결과
Name: nginx
Namespace: default
Labels: <none>
Annotations: <none>
Selector: app=my-app
Type: ClusterIP
IP Family Policy: SingleStack
IP Families: IPv4
IP: 10.96.192.111
IPs: 10.96.192.111
Port: <unset> 80/TCP
TargetPort: 80/TCP
Endpoints: 10.244.1.9:80,10.244.2.10:80,10.244.1.10:80
Session Affinity: None
Internal Traffic Policy: Cluster
Events: <none>
올라가 있는 파드를 지우거나 늘리거나 변경되면 Endpoints가 즉시 업데이트 된다.
Load Balancing
#03-svc-load-balancing.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deploy
spec:
selector:
matchLabels:
app: my-app
replicas: 3
template:
metadata:
labels:
app: my-app
spec:
terminationGracePeriodSeconds: 1
containers:
- name: nginx
image: vinsdocker/nginx-gke
ports:
- containerPort: 80
---
apiVersion: v1
kind: Service
metadata:
name: my-app
spec:
selector:
app: my-app
ports:
- port: 80
targetPort: 80
---
apiVersion: v1
kind: Pod
metadata:
name: demo-pod
spec:
terminationGracePeriodSeconds: 1
containers:
- name: demo
image: vinsdocker/util
args:
- "sleep"
- "3600"
kubectl apply -f 03-svc-load-balancing.yaml.yaml
클러스터 내부에서 파드끼리 통신하기 위한 demo-app과 로드밸런싱을 위한 service, my-app을 만든다.
이후 pod 내부에 접속한다.
kubectl exec -it demo-pod -- bash
이 후 요청을 보내보면 매번 다른 pod 이름을 보여주는것을 확인할 수 있다.
# 반복
root@demo-pod:/# curl my-app
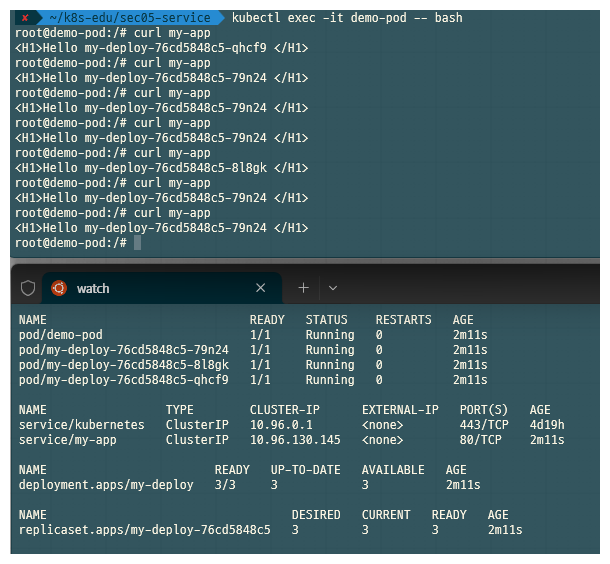
Service Types
타입 | 설명 |
ClusterIP | K8S 클러스터 내부에서 통신을 위한 타입이다. 클러스터 외부에서는 접근할 수 없다. 타입을 지정하지않으면 기본값이다. |
NodePort | 클러스터 외부에서 K8S 마스터/노드를 통해 특정 포트를 사용해서 접근할 수 있다. |
LoadBalancer | 외부에서 들어오는 트래픽을 수신하는데 사용된다. (AWS, GCP 같은 클라우드 업체에서 사용된다.) |
* 클러스터 외부의 노출은 ingress를 사용하며 이는 나중에 학습한다.
Kind cluster with extra port mapping
기존 클러스터를 삭제하고 추가 포트매핑을 한 클러스터를 생성해보자
kind delete cluster --name dev-cluster
아래처럼 파일을 생성하고 클러스터를 생성하자
kind: Cluster
apiVersion: kind.x-k8s.io/v1alpha4
name: dev-cluster
nodes:
- role: control-plane
extraPortMappings:
- containerPort: 30001
hostPort: 30001
protocol: TCP
- role: worker
- role: worker
kind create cluster --config [yaml 파일]
아래 명령어를 통해 port 매핑을 확인할 수 있다.
docker ps -a
# 결과
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS
NAMES
6e4af28b723c kindest/node:v1.31.2 "/usr/local/bin/entr…" 31 seconds ago Up 28 seconds 0.0.0.0:30001->30001/tcp, 127.0.0.1:45971->6443/tcp dev-cluster-control-plane
056d4e90bc99 kindest/node:v1.31.2 "/usr/local/bin/entr…" 31 seconds ago Up 28 seconds
dev-cluster-worker
76da068e1786 kindest/node:v1.31.2 "/usr/local/bin/entr…" 31 seconds ago Up 28 seconds
dev-cluster-worker2
Nodeport - Demo
# 05-nodeport-service.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-deploy
spec:
selector:
matchLabels:
app: my-app
replicas: 3
template:
metadata:
labels:
app: my-app
spec:
terminationGracePeriodSeconds: 1
containers:
- name: nginx
image: vinsdocker/nginx-gke
ports:
- containerPort: 80
---
apiVersion: v1
kind: Service
metadata:
name: my-app
spec:
type: NodePort
selector:
app: my-app
ports:
- port: 80
targetPort: 80
nodePort: 30001
kubectl apply -f 05-nodeport-service.yaml
watch 명령어로 확인해보면 NodePort가 있는것을 확인할 수 있고
아래 페이지로 들어가보면 접속이 되는것을 확인할 수 있다.
http://localhost:30001/
Rolling Update With Service
# 06-rolling-update-demo.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: order-service-deployment
spec:
minReadySeconds: 5
strategy:
type: RollingUpdate
rollingUpdate:
maxSurge: 0
maxUnavailable: 1
selector:
matchLabels:
app: order-service
replicas: 3
template:
metadata:
labels:
app: order-service
spec:
containers:
- name: order-service-container
image: vinsdocker/k8s-app:v1
ports:
- name: "app-port"
containerPort: 80
---
apiVersion: v1
kind: Service
metadata:
name: my-app
spec:
selector:
app: order-service
ports:
- port: 80
targetPort: 80
---
apiVersion: v1
kind: Pod
metadata:
name: demo-pod
spec:
terminationGracePeriodSeconds: 1
containers:
- name: demo
image: vinsdocker/util
args:
- "sleep"
- "3600"
kubectl apply -f 06-rolling-update-demo.yaml
위 파일을 생성하고 접속한다.
kubectl exec -it demo-pod -- bash
이후 아래 명령어를 쳐보면 V1이 나오는것을 확인할 수 있다.
for i in {1..1000}; do curl -s http://my-app | grep -o "<title>[^<]*" | tail -c+8; done
이때 빠르게 v2로 변경하고 적용하면 V1과 V2가 섞여서 나오는것을 확인할 수 있다.
그러다가 모두 V2로 변경되는것을 확인할 수 있다.
'Kubernetes' 카테고리의 다른 글
[Kubernetes] Probes (0) | 2024.12.19 |
---|---|
[Kubernetes] Namespace (0) | 2024.12.19 |
[Kubernetes] Deployment (0) | 2024.12.16 |
[Kubernetes] ReplicaSet (0) | 2024.12.12 |
[Kubernetes] Pod 기초 - 2 (1) | 2024.12.06 |